Flutter SDK Integration
Watch Video play_circleFollow the steps below to integrate the ByteBrew SDK or watch the tutorial video for a step by step walkthrough:
Import ByteBrew Flutter SDK
Add the ByteBrew Flutter SDK dependency below to your project's pubspec.yaml file. You can find the ByteBrew SDK on pub.dev
dependencies:
bytebrew_sdk: ^0.0.9
Go to your ByteBrew Dashboard
Go to your Game Settings page on the ByteBrew dashboard and locate your app keys listed on the dashboard. After finding your app keys, copy them.
Initialize ByteBrew with App Keys
Initialize ByteBrew using the code below at the beginning of your game to start capturing sessions and events.
Split the initialization by platform and input the corresponding app keys from the ByteBrew dashboard into their parameter fields.
if(Platform.isAndroid) {
ByteBrewSdk.initialize("APP_ID", "APP_KEY");
} else if(Platform.isIOS) {
ByteBrewSdk.initialize("APP_ID", "APP_KEY");
}
Exporting iOS Requirements
ByteBrew requires the following three iOS frameworks:
Security.Framework
AdServices.Framework
AdSupport.Framework
Implement these frameworks above in your final xcode project as frameworks.
Custom Events Tracking
Custom Events in ByteBrew enable you to deep dive across all of our extensive analytics dashboards. Continue below to learn how to integrate custom events.
Custom Events on ByteBrew allow for an unlimited number of key_name=value; sub-parameters under the custom event. For example, you can have a custom event called “level” that has a subparameter under the event named “levelnumber” and the values of that Level Number subparameter could be “1, 2, 3, 4, 5, etc.”. Additionally you could add in other subparameters for attributes inside the levels of your game, such as "powerups", to show the different equipment used in levels with parameter values "fireball, revive, doublepoints, etc.". The world is your oyster with custom events, so build as complex of an event system as you need for your game. To illustrate our custom event structure visually, see the following diagram:
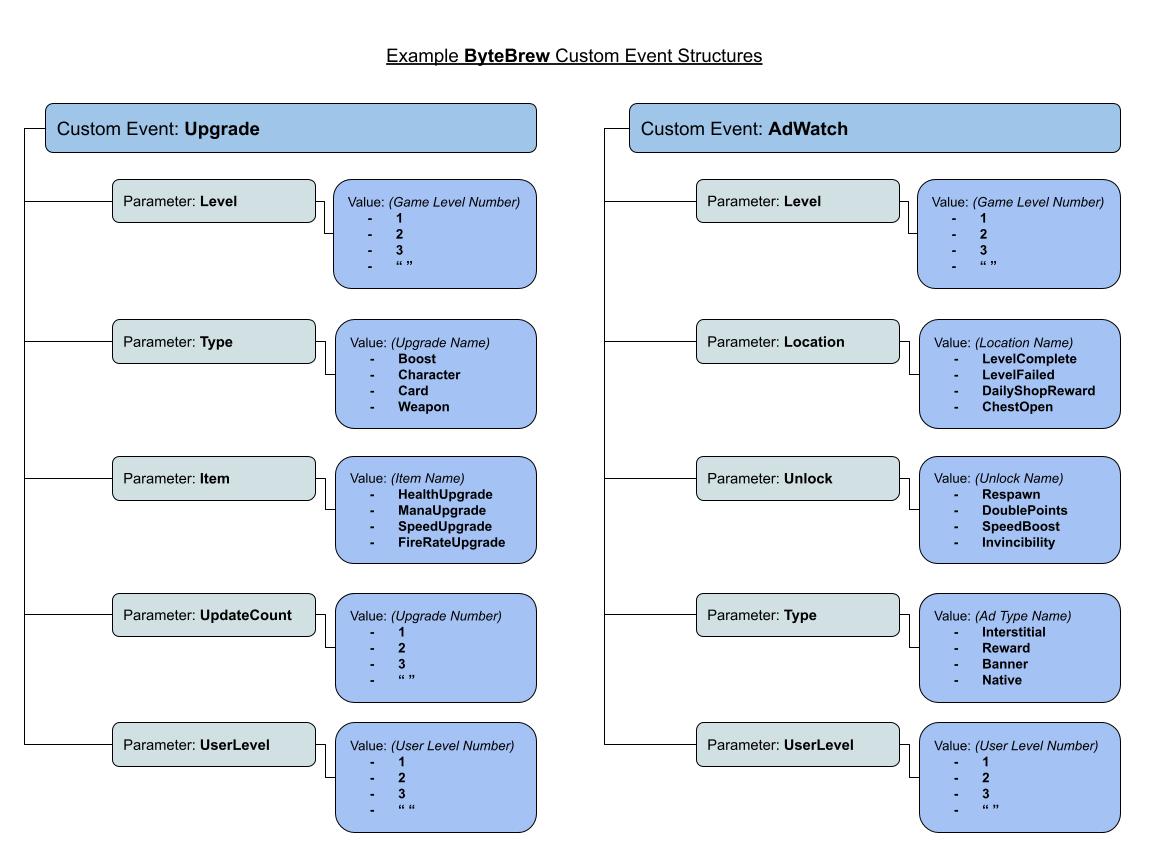
Custom Events
See samples below to see how to code Custom Events:
Custom event names and parameters that include special characters will not be processed on the dashboard. These events are unrecognized by ByteBrew and antipattern to ByteBrew's event structure.
Basic Custom Event:
//Basic Custom Event without any sub-parameters
ByteBrewSdk.newCustomEvent("eventName");
//Basic Custom Event with a sub-parameter
ByteBrewSdk.newCustomEventStringValue("CustomEventName", "key_name=value;");
//Examples
ByteBrewSdk.newCustomEventStringValue("GamePurchaseVC", "currency=GOLD;amount=500;character=viking;");
Dictionary Custom Event Tracking Method:
To easily add additional custom sub-parameters to an event with a dictionary method using string "key_name=value;" pairs shown below:
//Key Value format "key_name=value;"
//Custom Events can take multiple pairs, "key_name1=value1;key_name2=value2;key_name3=value3;"
ByteBrewSdk.newCustomEventStringValue("test_sub_params", "hello_world=yes;adwatch_count=1;");
Remote Configs & A/B Tests
Remote Configs allow you to make updates to your app remotely without having to update your game's app store. Adding remote configs to your games are a fantastic way to be agile and send updates or patches to players instantly. Remote Configs are also utilized for creating A/B Tests on the platform.
When planning to integrate Remote Configs, We recommend implementing remote configs throughout all parts of your game to allow you to tweak and tune as you analyze your game performance data. See how to implement remote configs in your game by following the steps below:
Loading the Remote Configs
To use Remote Configs, you must first call for the config to get updated. You can call the below code whenever you want to update the configs:
//Call the Action handler
ByteBrewSdk.loadRemoteConfigs().then((value) => {
});
//Check if the remote configs are ready and set
ByteBrewSdk.hasRemoteConfigs();
Retrieve the Configs
Once the action handler has finished, you can call the remote config method below:
//Call to get the key specific value and if the key doesn't exist it will return the default variable specified, like if the AB test user is in the control group
String item = await ByteBrewSdk.retrieveRemoteConfigValue("some_remote_setting", "10") ?? "None";
Push Notifications
Using ByteBrew you can send cross-platform push notifications to your players around the globe. To get started with push notifications follow the steps below.
Integrate Push Notifications
Integrating Push Notifications with ByteBrew is super simple and only requires one line of code after initializing the ByteBrew SDK. See the Push Notification integration code line below:
ByteBrewSdk.startPushNotifications();
Create Push Notification App on the ByteBrew Dashboard
Go to the Push Notification dashboard on ByteBrew and create a new Push Notification App. See our Push Notification Dashboard documentation for step by step walkthroughs about this.
Push Notification Android Manifest (Required Android Only)
<uses-permission android:name="android.permission.POST_NOTIFICATIONS"/>
Push Notification Xcode Export Additions (Required iOS Only)
For sending notifications to iOS devices, follow the additional required steps below on Xcode:
Add UserNotifications.framework
Add UserNotifications.framework to the frameworks and libraries in Xcode.
Add Capabilities
Press "+ Capability" and add the following capabilities listed in steps 3 and 4 below.

Add "Background Modes" and under that section, checkmark "Remote Notifications
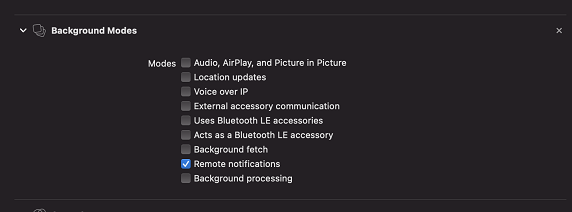
Add "Push Notifications"

Track Purchases
In ByteBrew SDK you can track all your game or apps in-app purchases to analyze your monetization performance on the dashboard. There are two ways to track purchases on ByteBrew. The first way is to use basic in-app purchase events. The second way is to use our real-time server-side purchase validation to validate real purchases vs. fraudulent transactions. See below to learn how to implement either method.
Track Basic In-App Purchases
To track in-app purchases in your game, use the below method:
ByteBrewSdk.trackInAppPurchase(store, currency, amount, itemID, category);
Track Validated Purchases
On ByteBrew, we offer a real-time server-side purchase validation to stop purchase fraud from client-side purchase receipt tampering that takes place in mobile games and apps. To utilize this validation you have two options: (1) a method with a callback to retrieve if a purchase was valid and (2) a method just to validate purchases without a callback. Follow below sections for code snippets for each function:
Purchase Validation with Callback
To track validated in-app purchases utilize the platform specific method. Using this method will create a purchase callback that will return a payload with the purchases details and verification status. See below for the Return Results and message definitions:
//JSON Format ex. string json = "{\"firstname\":\"john\", \"lastname\":\"doe\",\"age\":30}";
//Retrieve the iOS receipt from the purchase event that occurs. We will validate it server side so you can view valid purchases in you dashboard.
ByteBrewSdk.validateAppleInAppPurchase(store, currency, amount, itemID, category, receipt).then((value) =>
{
log(value!["purchaseProcessed"]) //bool telling if the purchase even went to the server to get checked
log(value!["isValid"]) //bool signaling if the purchase is real or fake
log(value!["message"]) //Message from the server telling the explaination behind the decision
log(value!["timestamp"])
});
//Retrieve the Android receipt and Signature from the purchase event that occurs. We will validate it server side so you can view valid purchases in you dashboard.
ByteBrewSdk.validateGoogleInAppPurchase(store, currency, amount, itemID, category, receipt, signature).then((value) =>
{
log(value!["purchaseProcessed"]) //bool telling if the purchase even went to the server to get checked
log(value!["isValid"]) //bool signaling if the purchase is real or fake
log(value!["message"]) //Message from the server telling the explaination behind the decision
log(value!["timestamp"])
});
Returned Results:
The following table are the possible returning results:
Returned Results Message:
In addition to the Return Result you will also recieve a message. See below for possible output messages and their definition:
"Validation Successful, real purchase.": This means that the message as a real purchase.
"Validation Failed, fake purchase.": This means that the purchase is fake or fraudulent.
"Error validating receipt, check game configs.": This message means that there might be an issue with the implementation. Check to make sure that the purchaseProcessed boolean is True and go to your game settings on the ByteBrew dashboard to update your Apple App Shared Secret and Google Play License Key.
Purchase Validation without Callback
To track in-app purchases using our validation system without looking for the purchase callback, utilize the platform specific method code snippets below:
//JSON Format ex. string json = "{\"firstname\":\"john\", \"lastname\":\"doe\",\"age\":30}";
//Retrieve the iOS receipt from the purchase event that occurs. We will validate it server side so you can view valid purchases in you dashboard.
ByteBrewSdk.trackAppleInAppPurchase(store, currency, amount, itemID, category, receipt);
//Retrieve the Android receipt and Signature from the purchase event that occurs. We will validate it server side so you can view valid purchases in you dashboard.
ByteBrewSdk.trackGoogleInAppPurchase(store, currency, amount, itemID, category, receipt, signature);
Ad Tracking Event
Using ByteBrew you can track your game's or app's ad events. Tracking Ad Events will enable you to see breakdowns of your ads in the monetization dashboard. See the code snippets below to learn how to track ad events:
// Record the Placement Type, Provider of the ad, ad unit name, and revenue of the impression
ByteBrewSdk.trackAdEvent(ByteBrewAdType.Interstitial, "Google AdMob", "Interstitial_Unit_Name", 0.00074145);
ByteBrewSdk.trackAdEvent(ByteBrewAdType.Reward, "Applovin Ads", "Reward_Unit_Name", 0.005562);
//Some ad event paramters can be ommited like so
ByteBrewSdk.trackAdEvent(ByteBrewAdType.Interstitial, "Google AdMob", "Interstitial_Unit_Name", "Interstitial_EndOfLevel", 0.0024145);
Data Attributes
Data attributes are tags in the SDK that you can give a user to define them more specifically on the dashboard when filtering. For example, you can use data attributes to tag to users to build player segments to send targeted push notifications. If you are looking to update values on users at certain locations of your game, then use Custom Events with subparamters to track that type of update. See the following code snippets for examples on how to use Data Attributes:
ByteBrewSdk.setCustomDataTagString("clicked", "yep");
ByteBrewSdk.setCustomDataTagInt("intItem", 2);
ByteBrewSdk.setCustomDataTagBool("boolItem", true);
ByteBrewSdk.setCustomDataTagDouble("doubleItem", 1.2);
Get User ID
GetUserID method in the SDK enables you to grab the current User ID of the user in your game. This is a handy method for finding the ID for a user you want to send a specific push notification to.
// Get the string userID
String user_id = await ByteBrewSdk.getUserID() ?? "N/A";
Stop Tracking Current User
In the ByteBrew SDK, you have the option to stop tracking any user in your game by calling the ByteBrew StopTracking method. This will immediately stop and disable any tracking for a user. As another alternative method to disabling tracking, you can build your own consent pop-up prompt screen in your game or app and delay the initialization of ByteBrew SDK until your user has consented/agreed to your prompt.
ByteBrewSdk.stopTracking();
SDK FAQs
ByteBrew has standardized events that are automatically tracked such as: install events and session events. These events are used throughout the platform to automatically calculate out-of-the-box metrics like: session length, playtime, retention and more.
here are several reasons ByteBrew does not cache offline player data such as:
(1) App File Size: For games tracking many parts of their games, users playing offline will generate a significant file size of cached data that will inflate the app size on the device.
(2) Reduce App Crashes: If the storage of offline data becomes too large, the device will not be able to open the app on lower end devices and cause a potential app crash. ByteBrew is designed to make sure those issues do not exist for your game.
(3) Data Accuracy Tampering: Cached data from historical game session will get uploaded into the system the next time the user comes back online. This mean malicious users can tamper with your app's data, leading to potential issues with accurate reporting for you to operate from.
ByteBrew supports Android 5.1 and above.
No, you can only use one push notification service or provider to send notifications at one time. However, if you are only using ByteBrew for our other services, then you can use another platform's push notifications SDK while still using ByteBrew SDK without any issues.
No, Push Notifications has it's own internal callback, so no other callback is necessary.
API Level 22 (Android 5.1)
A/B Tests deliver configs using your Remote Configs integration. Remote Configs delivered in milliseconds to your players after the ByteBrew SDK is initialized.
Yes, whether tracking in-app purchases or using Custom Events that send revenue parameters, the revenue you send should be in USD.